Personalized Conformal Prediction in Python: A Practical Guide
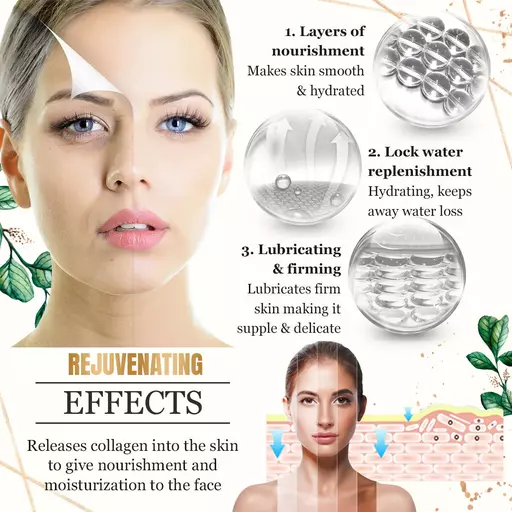
<!DOCTYPE html>
In the world of machine learning, making accurate predictions is crucial. However, understanding the uncertainty behind these predictions is equally important. Conformal prediction offers a robust framework to quantify this uncertainty, ensuring that predictions are not only accurate but also reliable. This blog post will guide you through implementing personalized conformal prediction in Python, providing practical insights and step-by-step instructions. Whether you're a data scientist, machine learning engineer, or enthusiast, this guide will help you master conformal prediction techniques tailored to individual needs.
What is Conformal Prediction?

Conformal prediction is a statistical framework that produces prediction sets with a guaranteed error rate. Unlike traditional methods that provide point estimates, conformal prediction offers a range of possible outcomes, ensuring a specified level of confidence. This makes it particularly useful in applications where understanding uncertainty is critical, such as healthcare, finance, and autonomous systems.
💡 Note: Conformal prediction is not limited to regression or classification tasks; it can be applied to any machine learning problem.
Why Personalized Conformal Prediction?

Personalized conformal prediction tailors the prediction sets to individual data points, accounting for specific characteristics or contexts. This approach enhances the relevance and accuracy of predictions, making it ideal for scenarios where one-size-fits-all models fall short. For instance, in personalized medicine, treatment recommendations can be customized based on a patient’s unique profile.
Getting Started: Tools and Libraries

To implement personalized conformal prediction in Python, you’ll need the following tools and libraries:
- Python: The primary programming language.
- Scikit-learn: For building and evaluating machine learning models.
- Nonconformist: A dedicated library for conformal prediction.
- NumPy and Pandas: For data manipulation and analysis.
📌 Note: Ensure you have Python 3.7 or higher installed for compatibility with the latest libraries.
Step-by-Step Implementation

Step 1: Install Required Libraries
Begin by installing the necessary libraries using pip:
pip install scikit-learn nonconformist numpy pandas
Step 2: Prepare Your Dataset
Load and preprocess your dataset. For personalized conformal prediction, ensure your data includes features that can be used for personalization. For example, in a healthcare dataset, patient demographics could be used for personalization.
Step 3: Train a Base Model
Train a machine learning model using Scikit-learn. This model will serve as the foundation for conformal prediction. For instance, you can train a random forest regressor:
from sklearn.ensemble import RandomForestRegressor
model = RandomForestRegressor()
model.fit(X_train, y_train)
Step 4: Apply Conformal Prediction
Use the Nonconformist library to apply conformal prediction. First, initialize a regressor and calibrate it using a calibration set:
from nonconformist.base import RegressorMixin
from nonconformist.cp import IcpRegressor
from nonconformist.nc import AbsErrorErrFunc
nc = IcpRegressor(RandomForestRegressor(), AbsErrorErrFunc())
nc.fit(X_calib, y_calib)
Step 5: Generate Prediction Sets
With the calibrated model, generate prediction sets for new data points. These sets will include a range of values with a specified confidence level:
prediction_sets = nc.predict(X_test, significance=0.1)
Checklist for Personalized Conformal Prediction

- Install required libraries: Scikit-learn, Nonconformist, NumPy, Pandas.
- Preprocess dataset with personalization features.
- Train a base machine learning model.
- Calibrate the conformal prediction model using a calibration set.
- Generate prediction sets with desired confidence levels.
By following these steps, you can effectively implement personalized conformal prediction in Python, enhancing the reliability and applicability of your machine learning models. (conformal prediction,machine learning,Python tutorials,data science,predictive modeling)
What is conformal prediction?
+Conformal prediction is a statistical framework that produces prediction sets with a guaranteed error rate, providing a range of possible outcomes with a specified confidence level.
How does personalized conformal prediction differ from standard conformal prediction?
+Personalized conformal prediction tailors prediction sets to individual data points, considering specific characteristics or contexts, whereas standard conformal prediction applies a uniform approach to all data points.
Which libraries are essential for implementing conformal prediction in Python?
+Essential libraries include Scikit-learn for model building, Nonconformist for conformal prediction, and NumPy/Pandas for data manipulation.